Android SDK Testing Guide
Started
- This document describes the usage of loplat SDK for debugging and testing.
- To test the app while it is running on a mobile device, make sure that it is set to "Location setting ON", "Always allow location permission", and "Do not optimize battery minimum".
NAVER Map Version 3
- NAVER Map needs to be applied to check the location recognition result.
- Refer to the NAVER Map application guide (http://docs.ncloud.com/ko/naveropenapi_v3/maps/android-sdk/v3/start.html).
The SDK version settings required for NAVER Map V3 are as follows.
compileSdkVersion 28
minSdkVersion 15
Apply and set up test mode
SDK version setting for test mode use
-
Declare
compileSdkVersion
,minSdkVersion
as below.-
To apply NAVER Map V3,
compileSdkVersion 28
declaration is required. -
In test mode,
minSdkVersion 19 (or higher)
declaration is required to operate the floating view shown on the screen.compileSdkVersion 28
minSdkVersion 19
-
Test Mode Required Permissions
-
The following rights must be allowed for test mode operation.
-
Location permission: To receive Wi-Fi scanning results and GPS (latitude and longitude) values
-
Write external storage: To write SDK operation logs to a file
-
Read account information: Used to send file logs by e-mail
-
Overlay Window : To display a floating view on the screen (refer to the picture below)
-
Appear on top
-
AndroidManifest.xml
in SDK is declared.
Use test mode
-
How to set up test mode
-
using maven
-
Add the code below to your project's top level
build.gradle
.allprojects {
repositories {
jcenter()
mavenCentral()
maven { url "https://maven.loplat.com/artifactory/plengi-debug"}
google()
}
} -
Add loplat SDK Dependency
dependencies {
implementation 'com.loplat:placeengine:2.1.1.6.1'
}
-
When using AAR
-
Copy the loplat android SDK AAR file to the library (libs) folder in the project folder
repositories {
flatDir { dirs 'libs' }
}
dependencies {
implementation fileTree(dir: 'libs', include: ['*.jar'])
}
-
Test mode configuration
The test mode consists of two screens: the SDK operation log screen and the location recognition result confirmation screen.
The test mode screen can be viewed by pressing the app launcher icon on the device screen.
SDK activity log screen
The SDK log screen consists of 4 function buttons and a list.
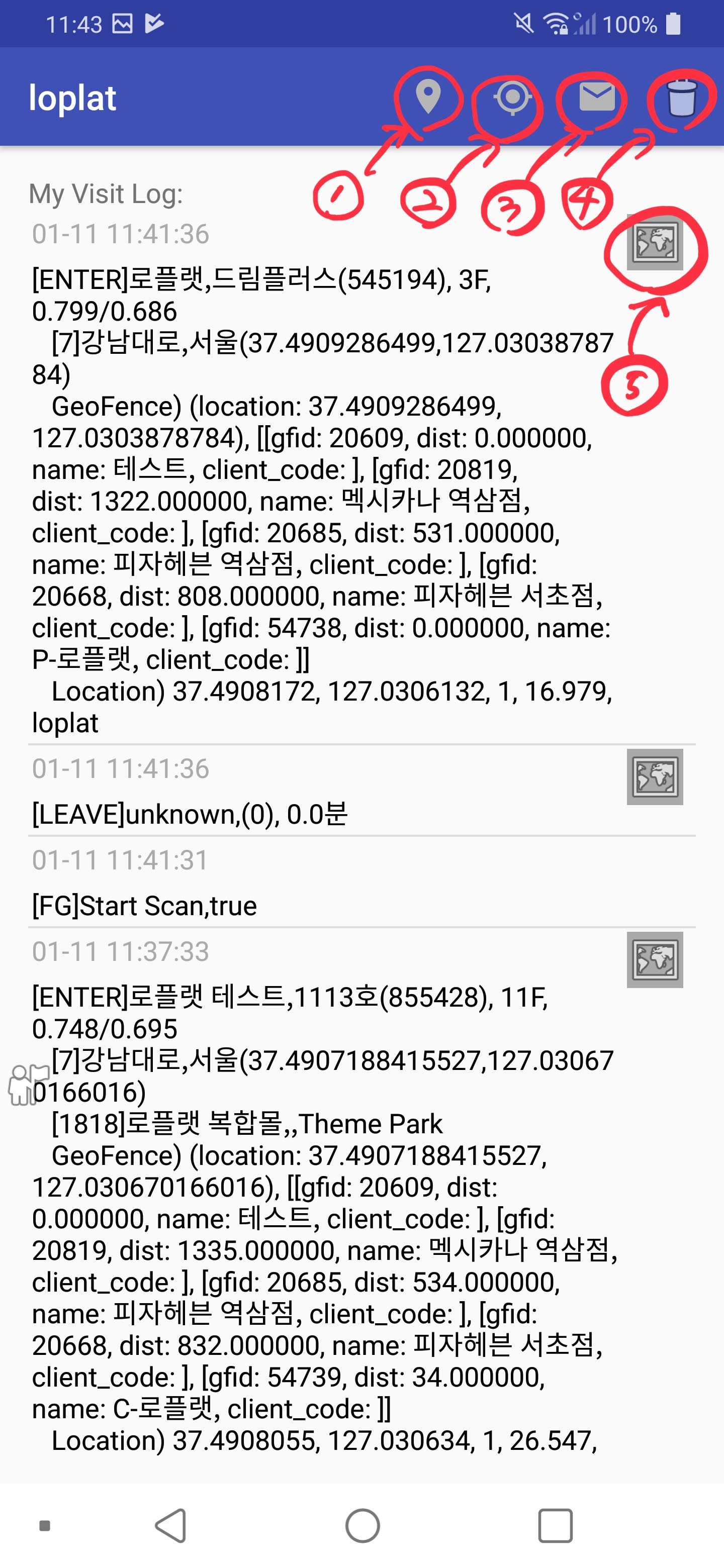
-
No. 1: Check your current location
- Check location through Wi-Fi scanning results from the current location
-
No. 2: Checking the current location based on LBS
- Check current location based on base station information
-
No. 3: Send log file via e-mail
- SDK operation logs can be sent to the desired e-mail address
-
No. 4: Deleting log files
-
No. 5: Check the location result
-
Log list
-
The log list screen consists of the SDK operation log and the map button to check the location of the place recognition result.
-
log description
[BG]/[FG]
: App state at the time of foreground and background wifi scanning- Event Log -
[ENTER]/[NEARBY]/[LEAVE]
: Recognition place result status value
[ENTER]Loplat,DreamPlus(12345),11F,0.806/0.700 //[Event Type(enter/nearby)] Place name, br/anch name/tag (place ID), floor information, recognition accuracy
[7] GangNam Dae-ro, Seoul(37.490718841, 127.03067071) //[Area ID], Commercial area (region) name (latitude, longitude) Location)
37.4906586, 127.030372, 1, 28.895, loplat //latitude, longitude, floor, accuracy, provider[LEAVE]Loplat,(1234),20분 // Logging of time spent at a place, place name, place ID, and time spent in order
-
Check the place recognition result
You can check the location of the place recognized through the SDK through the (Naver) map.
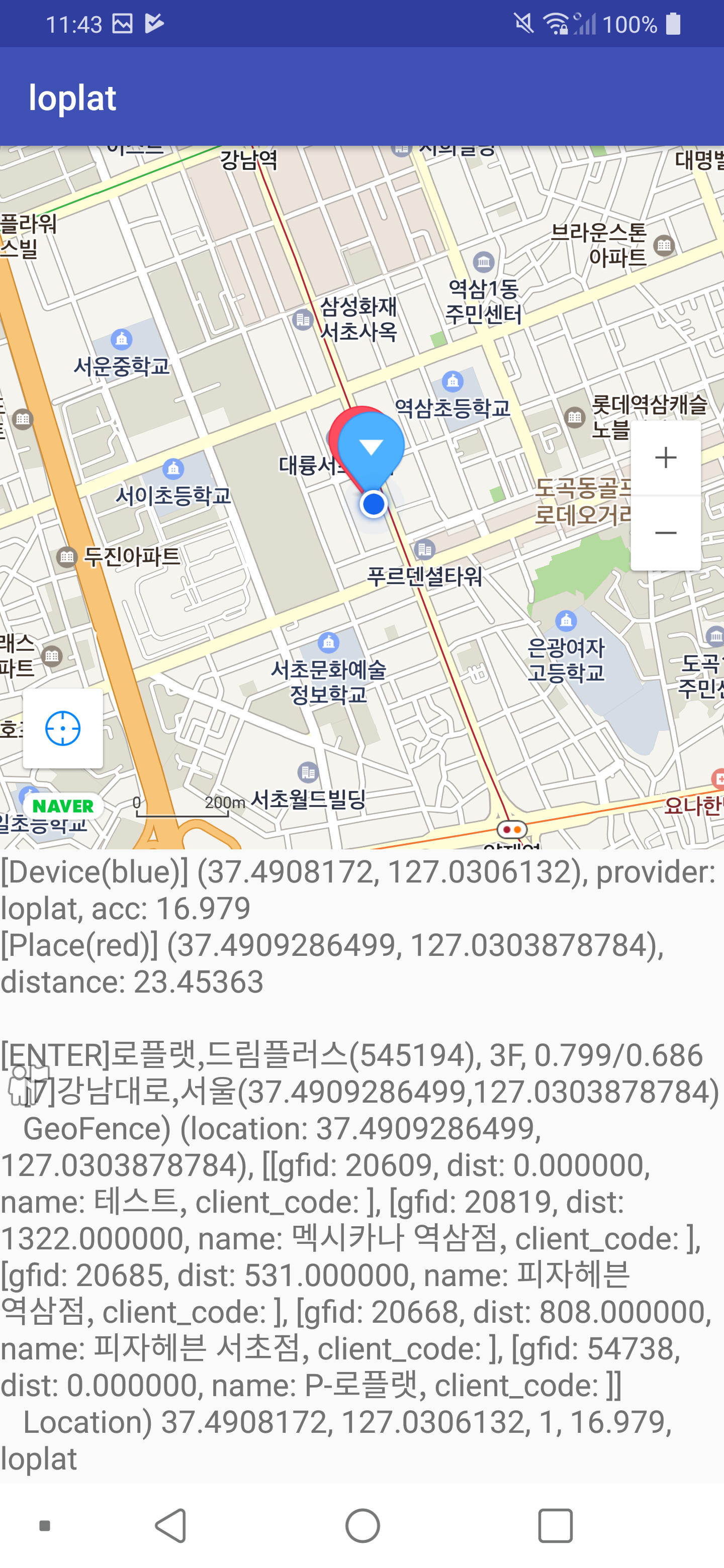
Test
Basic operation test based on WiFi
Place recognition scenario
Stays at store A for about 4 minutes -> A location recognition & location recognition event occurs
Store A (Location) (outside) Within 20m Stay for about 4 minutes -> Place A Nearby Event occurs
Places recognized as Nearby do not generate a Leave Event.
Stay at store A for about 4 minutes -> Recognize IN (ENTER) at location A -> Move away from location A (more than 20m) -> Leave event occurs after approximately 2 minutes
Stay at place A for about 4 minutes -> Recognize In (ENTER) at place A -> Move to place B -> Stay at place B for about 4 minutes -> A Occurrence of Place Leave Event, Recognition of Place B
Place recognition results
Before testing, please be sure to read the following information.
-
Store (Location) Recognition Result
-
In the case of store recognition
[ENTER/NEARBY]
: Place recognition result status value- ENTER: In-store (location) recognition success
- NEARBY: Location recognition within 20m of the place
-
store is not recognized (failed)
- Event is delivered (if there is no the first recognized log, see image), but commercial district, complex mall, geofence, etc., are recognized
- Location recognition failure -> When the requested location is not recognized as a store, commercial district, complex mall, or geofence
response fail: Location acquisition fail
checked by the log
-
If you leave the recognized store (place)
No leave events exist for commercial districts, complex malls, and geofences.
-
[LEAVE]
event occurs -
[leave event] log would look as follows
-
Event name, recognized store (location) name, br/anch name/tag (store ID), stay time in order
[LEAVE] Loplat, Hanwha Dream Plus (12359), 40 minutes
-
-
-
Commercial area recognition result
-
If the place requested for location recognition is requested is included in the commercial district, it passes as an event as follows.
-
[Commerce ID] Business name, city name (latitude and longitude of device at the time of location request)
[7] Gangnam-daero, Seoul (37.490719941, 127.030670166)
-
-
Complex mall recognition result
-
If the place requested for location recognition is within the complex mall, it passes as an event.
-
[Complex mall ID] Complex mall name, br/anch name, category
[1295]Loplat Complex Mall,,Theme Park
-
-
Geofence recognition result
- If the location requested for location recognition corresponds to the geofence, it delivers as an event like log 4 (See image).
- (location: requested latitude and longitude of the device), [[gfid, fence name, client_code], ....]
-
Latitude and longitude information of the device requesting location
- The latitude and longitude information of the device that requested location recognition (see image) is delivered to the event as shown in log 5.
- latitude, longitude, floor, accuracy, provider
check list
For the SDK to work without issues, it is necessary to check the list below in the table.
The SDK will not operate appropriately if applied differently from the items below. Please check after applying the SDK.
No. | Question | Y/N | comment |
---|---|---|---|
0 | Is the applied SDK Version the (latest) version written on the Loplat Developer website? | ||
1 | Are there any cases that SDK stops calls arbitrarily other than when the consent to the location agreement is withdrawn or when the loplat service expires? | Stop should not be called before start. If so, please discuss it with loplat. | |
2 | Is the location where SDK init is called written according to the guide? | Is init called when the application starts at first? For Android, check if setListener is precedent to init | |
3 | Does SDK init be called only when the user agrees to the location terms? | ||
4 | Is SDK start called only when the user agrees to the location agreement? | ||
5 | Are you using a different API than described in loplat Developer Page | If so, please discuss with loplat | |
6 | Personal information such as phone number and e-mail address must not be included in the echo_code field at init | In echo_code, enter a random number that can distinguish members. | |
7 | Isn't the same constant value entered in the echo_code at init? | If echo_code is not required, enter null. |
FAQ
- Please check the mobile hotspot OFF status.
"The SDK does not work when mobile hotspot (tethering) is turned on."
Since you don't get average Wi-Fi scan values, check the mobile hotspot status within the SDK.
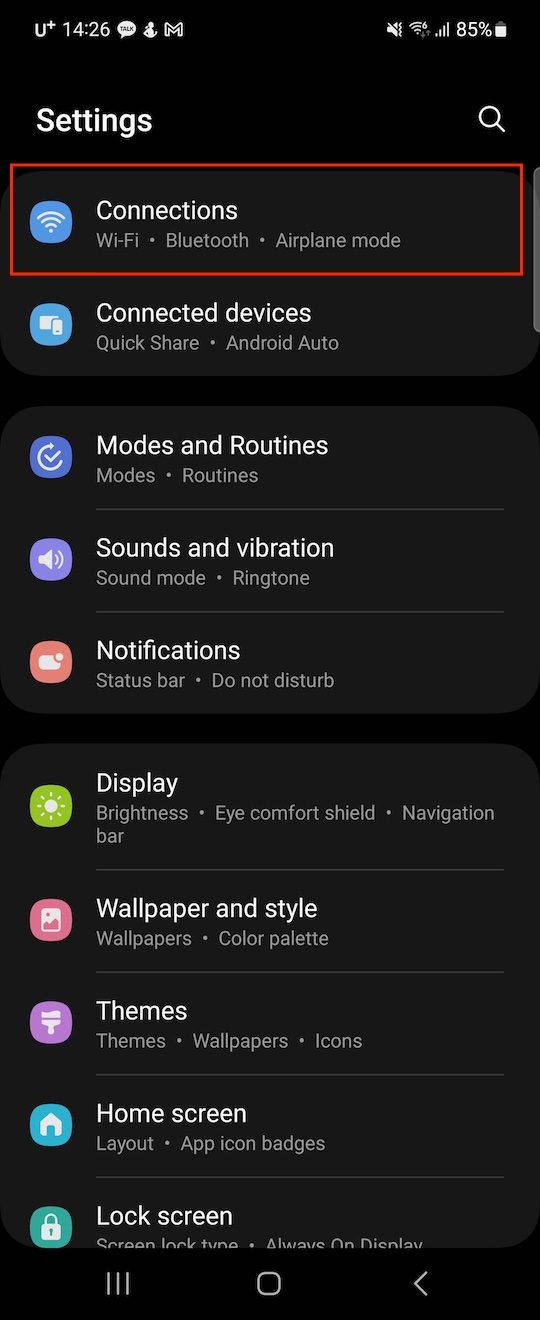
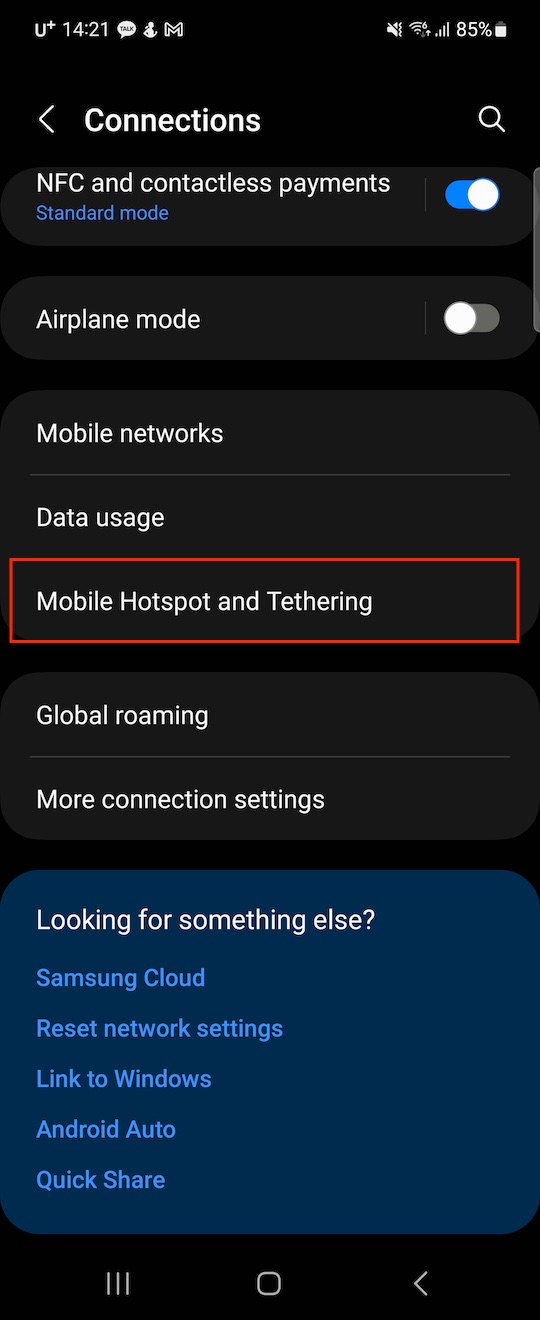
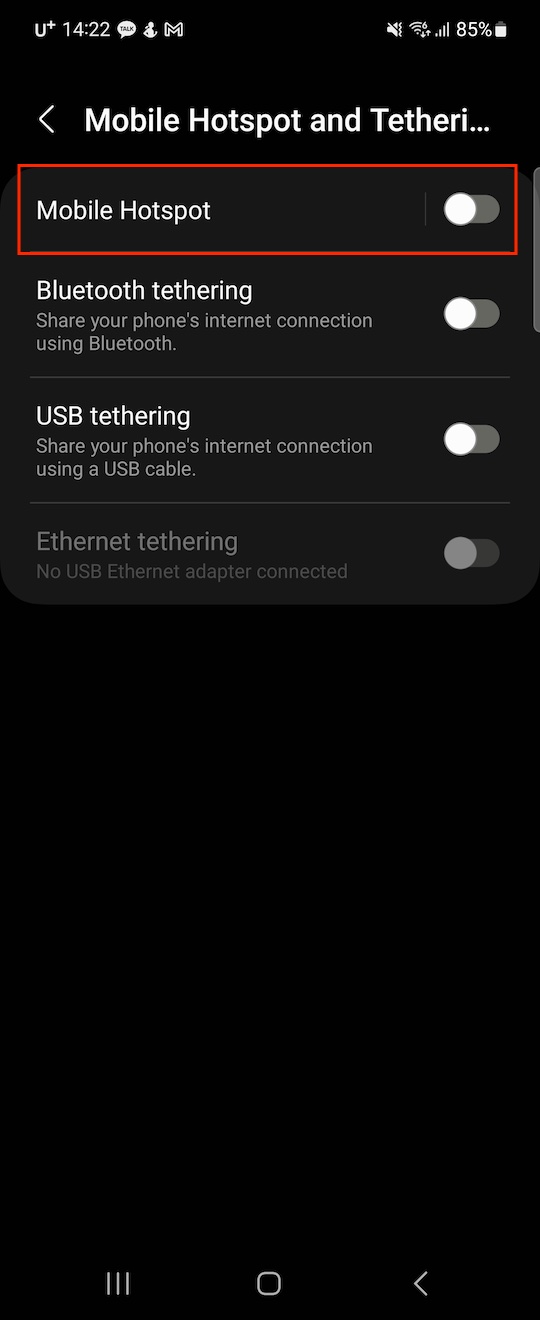
-
Allow location permission, Wi-Fi scanning availability
- How to check that Wi-Fi is always on (based on Samsung Galaxy phones)
- Settings -> Location -> Location services -> WiFi scanning (see image below)
-
How to check location permission status
- Settings -> Apps -> Select App -> Permissions -> Location -> Check location permission status
If you stay in one place for more than 4 minutes (more than 1 minute if the activity detection permission is ON), you can check the place recognition result.
However, please note that some differences depending on the Android OS version.
There is no problem with SDK operation, but high accuracy setting is recommended for accurate commercial area/geofence recognition.
Android 7.0
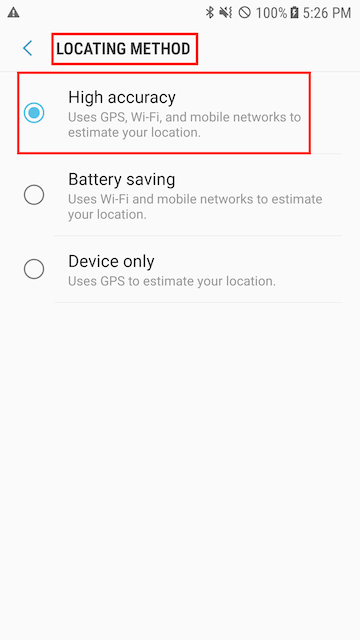
Android 13.0
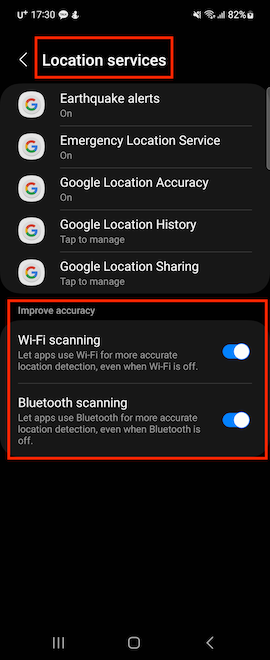